- 스탠퍼드 대학교의 Andrew Ng 교수님이 강의하신 ChatGPT Prompt Engineering for Developers 강의를 듣고 정리한 글입니다.
- 단순히 강의 내용을 번역한것이 아니라 내용을 이해한 뒤 정리하였고, 제 개인적인 생각은 +) 표시 뒤에 덧붙였습니다.
- 해당 글은 Iterative, Summarizing, Inferring까지의 내용을 담고 있으며, 이후 내용은 추후에 포스팅할 예정입니다.
- 훌륭한 강의이므로 아래 링크에서 직접 들어보시는 것을 추천드립니다.
ChatGPT Prompt Engineering for Developers
What you’ll learn in this course In ChatGPT Prompt Engineering for Developers, you will learn how to use a large language model (LLM) to quickly build new and powerful applications. Using the OpenAI API, you’ll...
www.deeplearning.ai
Iterative
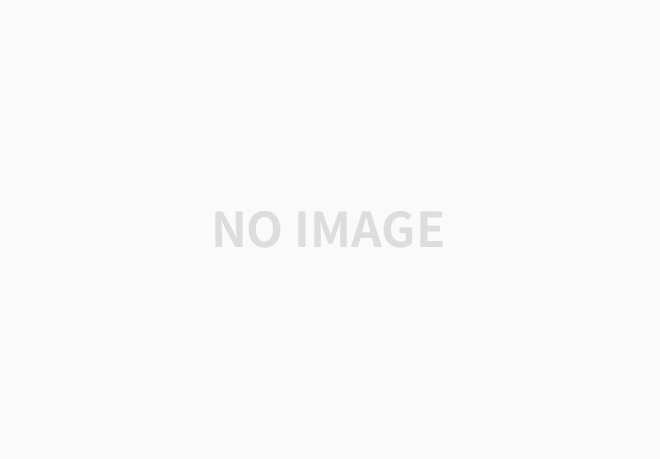
LLM을 활용하여 어플리케이션을 개발하기 위해 효율적인 프롬프트를 작성하기 위한 과정은 머신 러닝 모델을 개발하는 과정과 유사하다.
- 수행하고 싶은 task에 대한 아이디어를 떠올린다.
- 모델이 task를 수행하도록 프롬프트를 작성한다.
- 결과를 확인한다.
- 결과가 잘 나오지 않았다면, 프롬프트의 어느 부분에 문제가 있었는지 분석한다.
- 어플리케이션에 적합한 프롬프트가 만들어질 때까지 위 작업들을 반복적으로 수행한다.
I think there probably isn't a perfect prompt for everything under the sun. It's more important that you have a process for developing a good prompt for your specific application.
이 세상에 완벽한 프롬프트는 없다고 생각한다. 어플리케이션에 적합한 프롬프트를 개발하기 위한 프로세스가 중요한 것이다.
이전 강의와 동일한 코드로 OpenAI의 gpt-3.5-turbo 모델을 사용한다.
import openai
import os
from dotenv import load_dotenv, find_dotenv
_ = load_dotenv(find_dotenv()) # read local .env file
openai.api_key = os.getenv('OPENAI_API_KEY')
def get_completion(prompt, model="gpt-3.5-turbo"):
messages = [{"role": "user", "content": prompt}]
response = openai.ChatCompletion.create(
model=model,
messages=messages,
temperature=0, # this is the degree of randomness of the model's output
)
return response.choices[0].message["content"]
초기 프롬프트 작성
의자에 관한 정보를 바탕으로 상품 마케팅을 위한 설명 글을 생성하는 프롬프트를 작성해보자.
fact_sheet_chair = """
OVERVIEW
- Part of a beautiful family of mid-century inspired office furniture,
including filing cabinets, desks, bookcases, meeting tables, and more.
- Several options of shell color and base finishes.
- Available with plastic back and front upholstery (SWC-100)
or full upholstery (SWC-110) in 10 fabric and 6 leather options.
- Base finish options are: stainless steel, matte black,
gloss white, or chrome.
- Chair is available with or without armrests.
- Suitable for home or business settings.
- Qualified for contract use.
CONSTRUCTION
- 5-wheel plastic coated aluminum base.
- Pneumatic chair adjust for easy raise/lower action.
DIMENSIONS
- WIDTH 53 CM | 20.87”
- DEPTH 51 CM | 20.08”
- HEIGHT 80 CM | 31.50”
- SEAT HEIGHT 44 CM | 17.32”
- SEAT DEPTH 41 CM | 16.14”
OPTIONS
- Soft or hard-floor caster options.
- Two choices of seat foam densities:
medium (1.8 lb/ft3) or high (2.8 lb/ft3)
- Armless or 8 position PU armrests
MATERIALS
SHELL BASE GLIDER
- Cast Aluminum with modified nylon PA6/PA66 coating.
- Shell thickness: 10 mm.
SEAT
- HD36 foam
COUNTRY OF ORIGIN
- Italy
"""
prompt = f"""
Your task is to help a marketing team create a
description for a retail website of a product based
on a technical fact sheet.
Write a product description based on the information
provided in the technical specifications delimited by
triple backticks.
Technical specifications: ```{fact_sheet_chair}```
"""
response = get_completion(prompt)
print(response)
출력:
Introducing our stunning mid-century inspired office chair, the perfect addition \
to any home or business setting. Part of a beautiful family of office furniture, \
including filing cabinets, desks, bookcases, meeting tables, and more, \
this chair is available in several options of shell color and base finishes to \
suit your style. Choose from plastic back and front upholstery (SWC-100) or full \
upholstery (SWC-110) in 10 fabric and 6 leather options.
The chair is constructed with a 5-wheel plastic coated aluminum base and features \
a pneumatic chair adjust for easy raise/lower action. It is available with or \
without armrests and is qualified for contract use. The base finish options are \
stainless steel, matte black, gloss white, or chrome.
Measuring at a width of 53 cm, depth of 51 cm, and height of 80 cm, with a seat \
height of 44 cm and seat depth of 41 cm, this chair is designed for ultimate \
comfort. You can also choose between soft or hard-floor caster options and two \
choices of seat foam densities: medium (1.8 lb/ft3) or high (2.8 lb/ft3). \
The armrests are available in either an armless or 8 position PU option.
The materials used in the construction of this chair are of the highest quality. \
The shell base glider is made of cast aluminum with modified nylon PA6/PA66 \
coating and has a shell thickness of 10 mm. The seat is made of HD36 foam, \
ensuring maximum comfort and durability.
This chair is made in Italy and is the perfect combination of style and \
functionality. Upgrade your workspace with our mid-century inspired office chair \
today!
피드백 1: 내용이 너무 길어요
단어 또는 문장 또는 글자 수를 제한하여 내용을 줄여보자.
prompt = f"""
Your task is to help a marketing team create a
description for a retail website of a product based
on a technical fact sheet.
Write a product description based on the information
provided in the technical specifications delimited by
triple backticks.
Use at most 50 words.
Technical specifications: ```{fact_sheet_chair}```
"""
response = get_completion(prompt)
print(response)
출력:
Introducing our mid-century inspired office chair, perfect for home or business \
settings. Available in a range of shell colors and base finishes, with or without \
armrests. Choose from 10 fabric and 6 leather options for full or plastic \
upholstery. With a 5-wheel base and pneumatic chair adjust, it's both stylish and \
functional. Made in Italy.
프롬프트에 최대 50단어를 사용하라는 제한을 추가한 결과, 내용이 크게 줄어든 것을 확인할 수 있다.
실제로 단어 수를 확인해보면 55단어인 것을 확인할 수 있는데, 이는 LLM 모델의 특성상 정확한 단어의 개수를 맞추기 어렵기 때문이다.
+) decoder-only 모델인 ChatGPT의 특성상 <EOS> 토큰이 나올 때까지 문장이 생성되기 때문에 출력 단어의 개수를 정확하게 맞추기 어려운 것 같다.
피드백 2: 중요한 내용이 그게 아닌데..
모델이 생성한 답변이 우리가 의도한 내용에 초점을 두도록 프롬프트를 수정해보자.
prompt = f"""
Your task is to help a marketing team create a
description for a retail website of a product based
on a technical fact sheet.
Write a product description based on the information
provided in the technical specifications delimited by
triple backticks.
The description is intended for furniture retailers,
so should be technical in nature and focus on the
materials the product is constructed from.
At the end of the description, include every 7-character
Product ID in the technical specification.
Use at most 50 words.
Technical specifications: ```{fact_sheet_chair}```
"""
response = get_completion(prompt)
print(response)
출력:
Introducing our mid-century inspired office chair, perfect for home or business \
settings. With a range of shell colors and base finishes, and the option of \
plastic or full upholstery, this chair is both stylish and comfortable. \
Constructed with a 5-wheel plastic coated aluminum base and pneumatic chair \
adjust, it's also practical. Available with or without armrests and suitable for \
contract use. Product ID: SWC-100, SWC-110.
기술적인 내용과 제품을 구성하는 재료에 초첨을 두라는 내용과 설명 마지막 부분에 제품 ID를 포함하라는 내용을 프롬프트에 추가한 결과, 모델이 지시 사항에 알맞은 답변을 생성했다.
피드백 3: 웹 사이트에서 바로 사용할 수 있게 HTML로 만들어 줄 수 있나?
HTML로 답변을 생성하도록 프롬프트를 수정해보자.
prompt = f"""
Your task is to help a marketing team create a
description for a retail website of a product based
on a technical fact sheet.
Write a product description based on the information
provided in the technical specifications delimited by
triple backticks.
The description is intended for furniture retailers,
so should be technical in nature and focus on the
materials the product is constructed from.
At the end of the description, include every 7-character
Product ID in the technical specification.
After the description, include a table that gives the
product's dimensions. The table should have two columns.
In the first column include the name of the dimension.
In the second column include the measurements in inches only.
Give the table the title 'Product Dimensions'.
Format everything as HTML that can be used in a website.
Place the description in a <div> element.
Technical specifications: ```{fact_sheet_chair}```
"""
response = get_completion(prompt)
print(response)
출력:
<div>
<h2>Mid-Century Inspired Office Chair</h2>
<p>Introducing our mid-century inspired office chair, part of a beautiful family of office furniture that includes filing cabinets, desks, bookcases, meeting tables, and more. This chair is available in several options of shell color and base finishes, allowing you to customize it to your liking. You can choose between plastic back and front upholstery or full upholstery in 10 fabric and 6 leather options. The base finish options are stainless steel, matte black, gloss white, or chrome. The chair is also available with or without armrests, making it suitable for both home and business settings. Plus, it's qualified for contract use, ensuring its durability and longevity.</p>
<p>The chair's construction features a 5-wheel plastic coated aluminum base and a pneumatic chair adjust for easy raise/lower action. You can also choose between soft or hard-floor caster options and two choices of seat foam densities: medium (1.8 lb/ft3) or high (2.8 lb/ft3). The armrests are also customizable, with the option of armless or 8 position PU armrests.</p>
<p>The materials used in the chair's construction are of the highest quality. The shell base glider is made of cast aluminum with modified nylon PA6/PA66 coating, with a shell thickness of 10 mm. The seat is made of HD36 foam, ensuring maximum comfort and support.</p>
<p>Made in Italy, this mid-century inspired office chair is the perfect addition to any office space. Order yours today!</p>
<h3>Product IDs:</h3>
<ul>
<li>SWC-100</li>
<li>SWC-110</li>
</ul>
</div>
<table>
<caption>Product Dimensions</caption>
<tr>
<th>Dimension</th>
<th>Measurement (inches)</th>
</tr>
<tr>
<td>Width</td>
<td>20.87"</td>
</tr>
<tr>
<td>Depth</td>
<td>20.08"</td>
</tr>
<tr>
<td>Height</td>
<td>31.50"</td>
</tr>
<tr>
<td>Seat Height</td>
<td>17.32"</td>
</tr>
<tr>
<td>Seat Depth</td>
<td>16.14"</td>
</tr>
</table>
‘Product Dimensions’를 제목으로 갖는 테이블을 추가하고, 웹 사이트에서 사용할 수 있도록 HTML 포맷으로 답변을 생성하라는 내용을 프롬프트에 추가했다. 그 결과, 아래와 같은 내용을 보여주는 HTML 코드를 생성했다.

Guidelines의 내용을 바탕으로 우리가 원하는 지시 사항을 어떻게 해야 모델에게 명확하게 전달할 수 있을지, 또는 모델이 단계별 과정들을 밟아 나가며 우리가 원하는 답변을 생성할 수 있게끔 만들 수 있을지 다양하게 시도해봐야 한다.
Summarizing
판다 인형의 상품 리뷰 글을 gpt-3.5-turbo를 활용해 요약해보자.
prod_review = """
Got this panda plush toy for my daughter's birthday, \
who loves it and takes it everywhere. It's soft and \
super cute, and its face has a friendly look. It's \
a bit small for what I paid though. I think there \
might be other options that are bigger for the \
same price. It arrived a day earlier than expected, \
so I got to play with it myself before I gave it \
to her.
"""
단어 / 문장 / 글자 수 제한
prompt = f"""
Your task is to generate a short summary of a product \
review from an ecommerce site.
Summarize the review below, delimited by triple
backticks, in at most 30 words.
Review: ```{prod_review}```
"""
response = get_completion(prompt)
print(response)
출력:
Soft and cute panda plush toy loved by daughter, but a bit small for the price. \
Arrived early.
최대 30 단어로 상품 리뷰를 요약하라고 요청한 결과 상품 리뷰의 내용을 담은 요약문을 생성해주었다.
배송에 초점을 둔 요약문
요약문에서 중점적으로 다룰 주제를 지정하여 요청할 수 있다.
prompt = f"""
Your task is to generate a short summary of a product \
review from an ecommerce site to give feedback to the \
Shipping deparmtment.
Summarize the review below, delimited by triple
backticks, in at most 30 words, and focusing on any aspects \
that mention shipping and delivery of the product.
Review: ```{prod_review}```
"""
response = get_completion(prompt)
print(response)
출력:
The panda plush toy arrived a day earlier than expected, but the customer felt \
it was a bit small for the price paid.
상품의 배송 관련 내용들에 초점을 두라는 내용을 추가했더니 배송 관련된 내용 위주로 요약문이 생성되었다.
그러나 상품 리뷰에서 배송 관련된 내용만 보고 싶다면 위 요약문이 만족스럽지 못할 수 있다. 그럴때는 아래와 같은 방법으로 해결할 수 있다.
특정 내용 추출하기
원하는 내용 외의 내용이 필요 없다면 글을 요약해달라고 요청하는 대신 특정 내용을 추출해달라고 요청하면 된다.
prompt = f"""
Your task is to extract relevant information from \
a product review from an ecommerce site to give \
feedback to the Shipping department.
From the review below, delimited by triple quotes \
extract the information relevant to shipping and \
delivery. Limit to 30 words.
Review: ```{prod_review}```
"""
response = get_completion(prompt)
print(response)
출력:
The product arrived a day earlier than expected.
여러 개의 리뷰 요약하기
웹 사이트에 존재하는 수많은 리뷰들을 LLM을 통해 짧게 요약하면 다양한 리뷰들을 빠르게 훑어볼 수 있고, 효율적으로 상품에 대한 후기를 파악할 수 있다.
Inferring
LLM의 가장 큰 장점 중 하나는 감정 분석, 텍스트 추출과 같은 다양한 task들을 하나의 모델에서 처리할 수 있다는 점이다.
아래는 램프에 대한 리뷰 글이다.
lamp_review = """
Needed a nice lamp for my bedroom, and this one had \
additional storage and not too high of a price point. \
Got it fast. The string to our lamp broke during the \
transit and the company happily sent over a new one. \
Came within a few days as well. It was easy to put \
together. I had a missing part, so I contacted their \
support and they very quickly got me the missing piece! \
Lumina seems to me to be a great company that cares \
about their customers and products!!
"""
리뷰 감정 분석
prompt = f"""
What is the sentiment of the following product review,
which is delimited with triple backticks?
Give your answer as a single word, either "positive" \
or "negative".
Review text: '''{lamp_review}'''
"""
response = get_completion(prompt)
print(response)
출력:
positive
리뷰에 표현된 감정 추출
리뷰에서 표현된 감정들을 추출하기 위해서는 아래와 같이 프롬프트를 작성할 수 있다.
prompt = f"""
Identify a list of emotions that the writer of the \
following review is expressing. Include no more than \
five items in the list. Format your answer as a list of \
lower-case words separated by commas.
Review text: '''{lamp_review}'''
"""
response = get_completion(prompt)
print(response)
출력:
happy, satisfied, grateful, impressed, content
특정 정보 추출
리뷰에서 상품과 해당 상품을 만든 회사 정보를 추출하기 위해 아래와 같이 프롬프트를 작성할 수 있다.
prompt = f"""
Identify the following items from the review text:
- Item purchased by reviewer
- Company that made the item
The review is delimited with triple backticks. \
Format your response as a JSON object with \
"Item" and "Brand" as the keys.
If the information isn't present, use "unknown" \
as the value.
Make your response as short as possible.
Review text: '''{lamp_review}'''
"""
response = get_completion(prompt)
print(response)
출력:
{
"Item": "lamp",
"Brand": "Lumina"
}
위와 같은 방식으로 수많은 리뷰들에서 각각의 리뷰가 어떤 회사의 어떤 상품에 대한 리뷰인지 빠르게 파악할 수 있다.
여러 분석을 한번에 수행하기
prompt = f"""
Identify the following items from the review text:
- Sentiment (positive or negative)
- Is the reviewer expressing anger? (true or false)
- Item purchased by reviewer
- Company that made the item
The review is delimited with triple backticks. \
Format your response as a JSON object with \
"Sentiment", "Anger", "Item" and "Brand" as the keys.
If the information isn't present, use "unknown" \
as the value.
Make your response as short as possible.
Review text: '''{lamp_review}'''
"""
response = get_completion(prompt)
print(response)
출력:
{
"Sentiment": "positive",
"Anger": false,
"Item": "lamp with additional storage",
"Brand": "Lumina"
}
주제 추론
가상의 뉴스 기사에 대한 주제를 분석하는 프롬프트를 작성해보자.
story = """
In a recent survey conducted by the government,
public sector employees were asked to rate their level
of satisfaction with the department they work at.
The results revealed that NASA was the most popular
department with a satisfaction rating of 95%.
One NASA employee, John Smith, commented on the findings,
stating, "I'm not surprised that NASA came out on top.
It's a great place to work with amazing people and
incredible opportunities. I'm proud to be a part of
such an innovative organization."
The results were also welcomed by NASA's management team,
with Director Tom Johnson stating, "We are thrilled to
hear that our employees are satisfied with their work at NASA.
We have a talented and dedicated team who work tirelessly
to achieve our goals, and it's fantastic to see that their
hard work is paying off."
The survey also revealed that the
Social Security Administration had the lowest satisfaction
rating, with only 45% of employees indicating they were
satisfied with their job. The government has pledged to
address the concerns raised by employees in the survey and
work towards improving job satisfaction across all departments.
"""
prompt = f"""
Determine five topics that are being discussed in the \
following text, which is delimited by triple backticks.
Make each item one or two words long.
Format your response as a list of items separated by commas.
Text sample: '''{story}'''
"""
response = get_completion(prompt)
print(response)
출력:
government survey, job satisfaction, NASA, Social Security Administration, \
employee concerns
특정 주제에 해당하는 글인지 판단하기
관심 주제를 지정하고, 주어진 글이 해당 주제의 내용을 담고 있는지 판단해보자.
topic_list = [
"nasa", "local government", "engineering",
"employee satisfaction", "federal government"
]
prompt = f"""
Determine whether each item in the following list of \
topics is a topic in the text below, which
is delimited with triple backticks.
Give your answer as list with 0 or 1 for each topic.\
List of topics: {", ".join(topic_list)}
Text sample: '''{story}'''
"""
response = get_completion(prompt)
print(response)
출력:
nasa: 1
local government: 0
engineering: 0
employee satisfaction: 1
federal government: 1
+) 위와 같이 프롬프트를 작성하게 되면 모델이 생성한 출력이 일관성 있는 형식을 갖추지 않을 수 있기 때문에 production 환경에서 사용하기 적합하지 않다. 따라서 JSON 포맷으로 출력할 수 있도록 프롬프트를 아래와 같이 수정했다.
topic_list = [
"nasa", "local government", "engineering",
"employee satisfaction", "federal government"
]
prompt = f"""
Determine whether each item in the following list of \
topics is a topic in the text below, which
is delimited with triple backticks.
Give your answer as JSON format with topic in topic_list \
as the keys. Value of each keys should be 0 or 1.
List of topics: {", ".join(topic_list)}
Text sample: '''{story}'''
"""
response = get_completion(prompt)
print(response)
출력:
{
"nasa": 1,
"local government": 0,
"engineering": 0,
"employee satisfaction": 1,
"federal government": 1
}
'AI > Prompt Engineering' 카테고리의 다른 글
ChatGPT Prompt Engineering for Developers 정리 [3] (1) | 2023.05.03 |
---|---|
ChatGPT Prompt Engineering for Developers 정리 [1] (2) | 2023.04.30 |